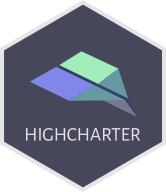
First steps on highcharter package
Joshua Kunst
2022-11-23
Source:vignettes/first-steps.Rmd
first-steps.Rmd
Introduction
We chart data, and data can come in different ways: numeric or character vectors, as time series objects, etc. but the most common object with data is a data frame. So, we’ll show how chart this type of object in highcharter.
Highcharter have two main functions to create a chart from data and
another to add data to an existing highchart
object.
-
hchart()
: A generic function which take an object (like vector, time series, data frames, likert object, etc) and return ahighchart
object (chart) -
hc_add_series()
: A generic function which add data to a existinghighchart
object depending the type (class) of the data.
There are a last function will be useful to chart data from data
frame. The functions is hcaes
which will define the
aesthetic mappings. This 3 functions are inspired in
ggplot2 package. So:
-
hchart()
works like ggplot2’sqplot
. -
hc_add_series()
works like ggplot2’sgeom_
s. -
hcaes()
works like ggplot2’saes
.
The main differences with ggplot2 are here we need the data and the aesthetics explicit in every highcharts functions.
First, show some data to work with.
## # A tibble: 6 × 11
## manufacturer model displ year cyl trans drv cty hwy fl class
## <chr> <chr> <dbl> <int> <int> <chr> <chr> <int> <int> <chr> <chr>
## 1 audi a4 1.8 1999 4 auto(l5) f 18 29 p compa…
## 2 audi a4 1.8 1999 4 manual(m5) f 21 29 p compa…
## 3 audi a4 2 2008 4 manual(m6) f 20 31 p compa…
## 4 audi a4 2 2008 4 auto(av) f 21 30 p compa…
## 5 audi a4 2.8 1999 6 auto(l5) f 16 26 p compa…
## 6 audi a4 2.8 1999 6 manual(m5) f 18 26 p compa…
In general we’ll use this structure to get a chart:
hchart(<data.frame>, <type_of_chart>, hcaes(<aesthetics>), ...)
-
<data.frame>
will be the data to chart. -
<type_of_chart>
is a string to specify the type of chart. This value can be: line, spline, area, heatmap, treemap, etc. -
<aesthetics>
is the mapping to use for plot. -
...
are other parameters to configure the chart.
So, a basic example would be:
Exercising the basics
Let’s try other charts to get familiar with the syntax.
data(economics_long, package = "ggplot2")
economics_long2 <- dplyr::filter(economics_long, variable %in% c("pop", "uempmed", "unemploy"))
head(economics_long2)
## # A tibble: 6 × 4
## date variable value value01
## <date> <chr> <dbl> <dbl>
## 1 1967-07-01 pop 198712 0
## 2 1967-08-01 pop 198911 0.00164
## 3 1967-09-01 pop 199113 0.00330
## 4 1967-10-01 pop 199311 0.00492
## 5 1967-11-01 pop 199498 0.00646
## 6 1967-12-01 pop 199657 0.00777
Now, a heatmap and a treemap.
Extra parameters
You can add other parameters to add options to the data series. This options can modify the names of each series/group. These names are used in the legend as well the tooltip. You can modify the colors and a lot of properties.
mpgman2 <- count(mpg, manufacturer, year)
hchart(
mpgman2,
"bar",
hcaes(x = manufacturer, y = n, group = year),
color = c("#7CB5EC", "#F7A35C"),
name = c("Year 1999", "Year 2008"),
showInLegend = c(TRUE, FALSE) # only show the first one in the legend
)
Highcharts has a very complete API with a lot of options. You can see every option in action in https://api.highcharts.com/highcharts/plotOptions.series.
A more advanced example
Using the broom
package is really nice due the you can
transform models to tidy data:
library(broom)
modlss <- loess(dist ~ speed, data = cars)
fit <- arrange(augment(modlss), speed) |>
mutate(.se = predict(modlss, se = TRUE)$se.fit)
head(fit)
## # A tibble: 6 × 5
## dist speed .fitted .resid .se
## <dbl> <dbl> <dbl> <dbl> <dbl>
## 1 2 4 5.89 -3.89 9.89
## 2 10 4 5.89 4.11 9.89
## 3 4 7 12.5 -8.50 4.99
## 4 22 7 12.5 9.50 4.99
## 5 16 8 15.3 0.719 4.55
## 6 10 9 18.4 -8.45 4.31
In this case we’ll chart the original data first and store in a
variable called hc
(for highcharts):
hc <- hchart(
cars,
type = "scatter",
hcaes(x = speed, y = dist),
name = "Speed and Stopping Distances of Cars",
showInLegend = TRUE
)
hc
We can add more series to chart (like the layers in ggplot world)
using the function hc_add_series
. The parameters in this
functions are similar to the hchart
:
hc_add_series(<highcarter_object>, <data.frame>, <type_of_chart>, hcaes(<aesthetics>), ...)
# or with the pipe:
<highcarter_object> |> hc_add_series(<data.frame>, <type_of_chart>, hcaes(<aesthetics>), ...)
In this case we will add 2 groups of series. The 1st to add will be
the spline then the arearange. So we’ll use hc_add_series
2
times.
qtint <- qt(0.975, predict(modlss, se = TRUE)$df)
hc |>
hc_add_series(
fit,
type = "spline",
hcaes(x = speed, y = .fitted),
name = "Fit",
id = "fit", # this is for link the arearange series to this one and have one legend
lineWidth = 1,
showInLegend = TRUE
) |>
hc_add_series(
fit,
type = "arearange",
name = "SE",
hcaes(x = speed, low = .fitted - qtint*.se, high = .fitted + qtint*.se),
linkedTo = "fit", # here we link the legends in one.
showInLegend = FALSE,
color = hex_to_rgba("gray", 0.2), # put a semi transparent color
zIndex = -3 # this is for put the series in a back so the points are showed first
)
Example of data from https://stackoverflow.com/questions/22717930/how-to-get-the-confidence-intervals-for-lowess-fit-using-r.
What now?
Since you know the basics now it could be interesting:
- Go motivate yourself with the
vignette("showcase")
and observe everything that can be done with the highcharter package. - Learn to configure graphics using the implemented API in
vignette("highcharts-api")
. - See all chart you can make in
vignette("highcharts")
.